It´s Viktor Dev
I am Viktor
How to Use the MVC Design Pattern in Web Development
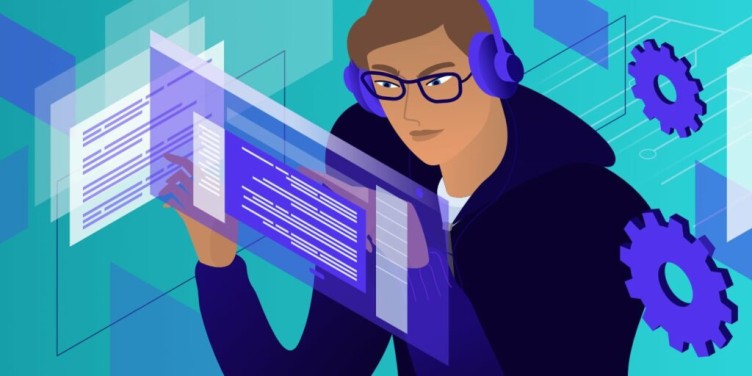
Web development requires an organized and maintainable structure to ensure project efficiency and scalability. This is where the Model-View-Controller (MVC) design pattern comes into play. MVC is an architecture that separates business logic, presentation, and user interaction into three distinct components. We will delve into the MVC design pattern in detail and explore how to effectively use it in web development.
What is the MVC Design Pattern?
The MVC design pattern is a methodology that divides an application into three main components: the Model, the View, and the Controller. Each component has a specific responsibility and works together to achieve a clear separation of concerns and more modular and reusable code.
The Model represents the business logic and underlying data of the application. It handles interactions with the database, performs calculations, and manages domain logic.
The View is the presentation layer of the application. It displays data to the user and handles visual interaction. It can be an HTML page, a graphical interface, or any element that presents information to the user.
The Controller acts as an intermediary between the Model and the View. It receives user requests, processes the corresponding logic, and updates the Model and View as needed.
Implementation Examples of MVC in Frameworks and Programming Languages
a) MVC Example in Ruby on Rails:
Ruby on Rails is a popular web development framework that natively uses the MVC design pattern. Let's see how it's implemented in this context:
Model: In Ruby on Rails, models are classes that represent database tables. For instance, if we have a "Users" table, the corresponding model would be the "User" class that interacts with that table.
View: In Ruby on Rails, views are .html.erb files that combine HTML and Ruby code to display data to the user.
Controller: Controllers in Ruby on Rails are classes that handle user requests and manage application logic.
b) MVC Example in Laravel (PHP):
Laravel is another popular framework that uses the MVC design pattern. Here's how it's implemented in this case:
Model: In Laravel, models represent database tables and contain business logic related to that data.
View: In Laravel, views are .blade.php files that combine HTML and PHP code to present data to the user.
Controller: Controllers in Laravel handle user requests, process application logic, and update the model and corresponding view.
Benefits of Using the MVC Design Pattern in Web Development
Implementing the MVC design pattern in web development offers several benefits:
- Separation of Concerns: MVC clearly separates business logic, presentation, and user interaction into independent components, facilitating maintenance and scalability.
- Code Reusability: Dividing the application into layers promotes code reuse. For example, models can be used by multiple controllers, and views can be reused in different contexts.
- Readability and Maintainability: MVC's clear and organized structure enhances code understanding and maintenance over time.
- Testing Ease: Separating business logic simplifies unit and integration testing, increasing overall system reliability.
Implementing the Model-View-Controller (MVC) design pattern in web development is crucial for achieving an organized and maintainable structure. By separating business logic, presentation, and user interaction into distinct components, MVC enhances project efficiency and scalability. Using practical examples from frameworks like Ruby on Rails and Laravel, we've demonstrated how this architecture is implemented in different contexts. With benefits such as separation of concerns, code reusability, and testing ease, MVC becomes a valuable tool for web developers seeking more modular, readable, and maintainable code.